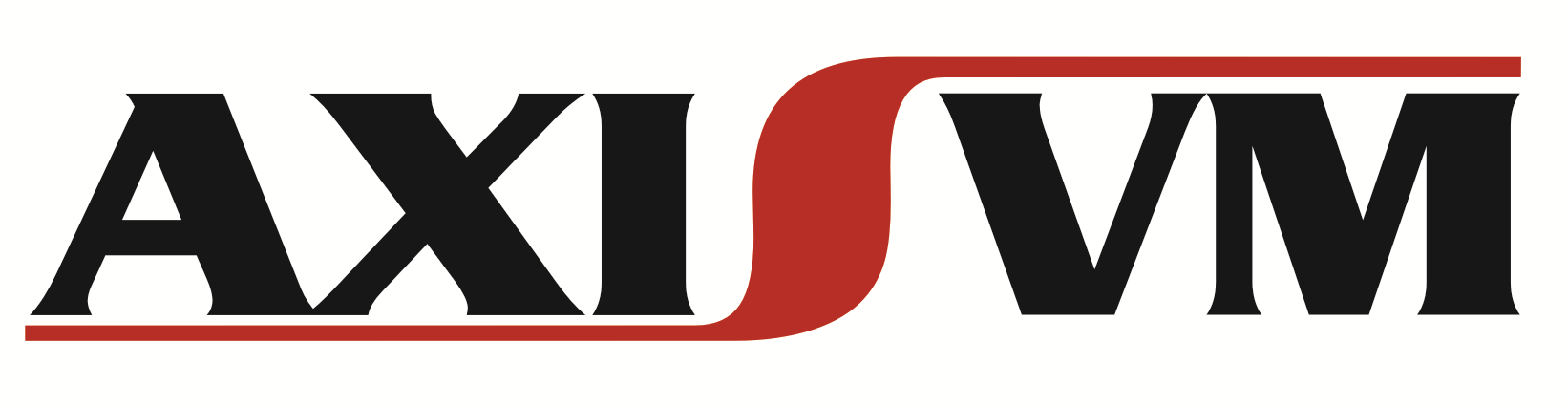
PyAxisVM - The official Python package for AxisVM#
Version: 1.2.2
Useful links: Getting Started | User Guide | Gallery | API Reference | Tips and Tricks | Source Repository
The PyAxisVM project offers a high-level interface to AxisVM, making its operations available directly from Python. It builds on top of Microsoft’s COM technology and supports all the features of the original AxisVM COM type library.
Highlights#
Build, manipulate and analyse AxisVM models.
Find better solutions with iterative methods.
Combine the power of AxisVM with your favourite Python libraries you already love.
Build extension modules.
And many more neither of us know about yet.
On top of that, PyAxisVM enhances the type library with Python’s slicing mechanism, context management and more, that helps you to write clean, concise, and readable code.
Installation#
You can install the project from PyPI with pip:
$ pip install axisvm
Contents#
Getting Started
The getting started guide helps you to make the first steps to get to know the library.
User Guide
The user guide contains a basic introduction to the main concepts through simple examples.
API Reference
The reference guide contains a detailed description of the functions, modules, and objects included in the library. The reference describes how the methods work and which parameters can be used. It assumes that you have an understanding of the key concepts.
Examples Gallery
A gallery of examples that illustrate uses cases that involve some kind of visualization.